Vue3对接ChatGPT4接口(打字机效果)
本篇文章将介绍如何在 Vue3 中实现与 ChatGPT4 API 的对接。具体的后端 API 对接方式,还请参考这篇文章:《对接ChatGPT3.5/4的API实现打字机效果》。
Vue3代码
<script setup lang="ts">
import { ref } from 'vue'
import Text from './Text.vue'
import { Message } from './GPTComponentType'
import { defineComponent } from 'vue'
defineComponent({
name: 'GPT'
})
const props = defineProps({
url: {
type: String,
required: true
},
auth: {
type: String,
required: true
}
})
const output = ref<string>('')
let abortController: AbortController = new AbortController()
let shouldCancelReadChunk: boolean[] = []
const getGPTAnswer = async (messages: Message[]) => {
abortController.abort() // 取消当前请求
abortController = new AbortController() // 创建新的 AbortController
// 将 shouldCancelReadChunk 的值设置为 true
shouldCancelReadChunk.forEach((_, idx) => {
shouldCancelReadChunk[idx] = true
})
shouldCancelReadChunk.push(false)
let index = shouldCancelReadChunk.length - 1
try {
let result = ''
output.value = '请求中...'
const res = await fetch(props.url, {
method: "POST",
body: JSON.stringify({
auth: props.auth,
messages
}),
headers: {
"Content-Type": "application/json"
},
signal: abortController.signal // 绑定 AbortSignal 到请求
})
if (res.body == null) {
return
}
const reader = res.body.getReader()
const decoder = new TextDecoder("utf-8")
const readChunk = async (): Promise<void> => {
// 在循环内检查 shouldCancelReadChunk 的值
if (shouldCancelReadChunk[index]) {
// 如果需要取消,则提前返回并停止执行 readChunk
return
}
const { value, done } = await reader.read()
if (!done) {
const dataString = decoder.decode(value)
const lines = dataString.trim().split("data:")
for (const line of lines) {
// 在循环内检查 shouldCancelReadChunk 的值
if (shouldCancelReadChunk[index]) {
// 如果需要取消,则提前返回并停止执行
return
}
if (line != '') {
const text = line.replace("data:", "").trim()
try {
const data = JSON.parse(text)
if (data.choices[0].delta.content) {
result += data.choices[0].delta.content
output.value = result
}
if (data.choices[0].finish_reason === 'length') {
messages.push({
role: 'assistant',
content: result
})
await getGPTAnswer(messages)
return
} else if (data.choices[0].finish_reason === 'stop') {
output.value = result
return
}
} catch (error: any) {
if (error && error.code && error.code === 20) {
console.log('请求被用户终止')
return
}
if (text.trim() === '[DONE]') {
output.value = result
return
}
console.error(error)
return
}
}
}
await readChunk() // 如果没有触发结束标识,则继续读取
} else {
console.log('done')
}
}
await readChunk()
} catch (error: any) {
if (error.name === 'AbortError') {
console.log('Request was canceled')
} else {
console.error(error)
}
}
}
defineExpose({
getGPTAnswer
})
</script>
<template>
<div class="gpt">
<Text :text="output" />
</div>
</template>
<style>
.gpt {
width: 100%;
}
</style>
GPTComponentType.ts:
import { ComponentPublicInstance } from 'vue'
export type Message = {
role: 'user' | 'assistant'
content: string
}
export type GPTComponentType = ComponentPublicInstance<{
getGPTAnswer: (messages: Message[]) => Promise<void>
}>
实现原理:
- 使用 Vue 3 的 Composition API,我们导入了必要的依赖和定义了组件、props 以及响应式变量。
- 为了处理多个请求并能够取消正在进行的请求,我们使用了
AbortController
。 - 在
getGPTAnswer
函数中,通过 POST 请求发送用户消息,并将结果保存在一个可观察的变量output
中。 - 当从服务器接收到数据时,我们将每条消息解析为 JSON 格式,检查是否有输出内容,如果有,则将其添加到
result
变量中。 - 如果在解析过程中发生错误,我们会在控制台上显示错误信息。
- 为了在请求过程中实现取消功能,我们使用了一个名为
shouldCancelReadChunk
的数组来跟踪每个请求的取消状态。当数组中的值为真时,请求将被取消。
代码处理了重复调用getGPTAnswer
方法的情况,当重复调用时会中止上一轮未完成的请求。
渲染Markdown
需要特别注意的是,上述代码并未对Markdown做出渲染,可以在 Text
组件中使用开源库 'markdown-it' 和 'highlight.js' 来实现实时 Markdown 渲染。由于Text.vue
的实现相对简单,在此不再赘述。
调用示例
Vue3代码:
<script setup lang="ts">
import { ref } from 'vue'
import GPT from './components/GPT.vue'
import type { GPTComponentType, Message } from './components/GPTComponentType'
// (messages: any[]) => Promise<void>
const gpt = ref<GPTComponentType | null>(null)
const url = 'https://example.com'
const auth = ''
const content = ref('')
const callGPTAnswer = async () => {
const messages: Message[] = [
// 您可以根据需要添加您自己的消息列表
{
role: 'user',
content: content.value
}
]
await gpt.value!.getGPTAnswer(messages)
}
</script>
<template>
<div class="container">
<textarea v-model="content" rows="8"></textarea>
<button @click="callGPTAnswer">获取 GPT 回答</button>
<GPT ref="gpt" :url="url" :auth="auth" />
</div>
</template>
通过以上步骤,您可以在 Vue3 项目中实现 ChatGPT4 API 的对接。
除特别注明外,本站所有文章均为原创。原创文章均已备案且受著作权保护,未经作者书面授权,请勿转载。
打赏
交流区
暂无内容
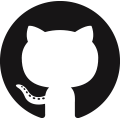
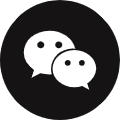
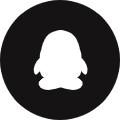
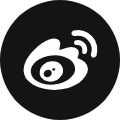
老师你好,我希望能用一个openwrt路由器实现IPv4和IPv6的桥接,请问我该如何实现?我尝试了直接新增dhcpv6的接口,但是效果不甚理想(无法成功获取公网的ipv6,但是直连上级路由的其他设备是可以获取公网的ipv6地)
你好
,为什么我这里是0039 813C 0600 0075 16xx xx xx,只有前6组是相同的,博客中要前8位相同,这个不同能不能照着修改呢?我系统版本是Win1124H2
大神你好,win11专业版24h2最新版26100.2033,文件如何修改?谢谢
win11专业版24h2最新版26100.2033,Windows Feature Experience Pack 1000.26100.23.0。C:\Windows\System32\termsrv.dll系统自带的这个文件,39 81 3C 06 00 00 0F 85 XX XX XX XX 替换为 B8 00 01 00 00 89 81 38 06 00 00 90。仍然无法远程连接。原来是win11 21h2系统,是可以远程链接的。共享1个主机,2个显示器,2套键鼠,各自独立操作 各自不同的账号,不同的桌面环境。
博主,win11专业版24h2最新版,C:\Windows\System32\termsrv.dll系统自带的这个文件,找不到应该修改哪个字段。我的微信:一三五73二五九五00,谢谢